Add OpenBSD RAM stats
This commit is contained in:
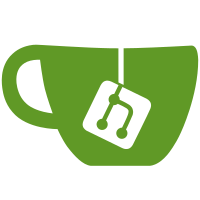
committed by
Aaron Marcher
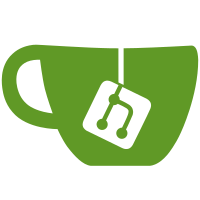
parent
faa52bdcc0
commit
88bf05e4a3
@@ -1,9 +1,9 @@
|
|||||||
/* See LICENSE file for copyright and license details. */
|
/* See LICENSE file for copyright and license details. */
|
||||||
#if defined(__linux__)
|
|
||||||
#include <stdio.h>
|
#include <stdio.h>
|
||||||
|
|
||||||
#include "../util.h"
|
#include "../util.h"
|
||||||
|
|
||||||
|
#if defined(__linux__)
|
||||||
const char *
|
const char *
|
||||||
ram_free(void)
|
ram_free(void)
|
||||||
{
|
{
|
||||||
@@ -51,4 +51,78 @@ ram_used(void)
|
|||||||
bprintf("%f", (float)(total - free - buffers - cached) / 1024 / 1024) :
|
bprintf("%f", (float)(total - free - buffers - cached) / 1024 / 1024) :
|
||||||
NULL;
|
NULL;
|
||||||
}
|
}
|
||||||
|
#elif defined(__OpenBSD__)
|
||||||
|
#include <sys/types.h>
|
||||||
|
#include <sys/sysctl.h>
|
||||||
|
#include <stdlib.h>
|
||||||
|
#include <unistd.h>
|
||||||
|
|
||||||
|
inline int
|
||||||
|
load_uvmexp(struct uvmexp *uvmexp)
|
||||||
|
{
|
||||||
|
int uvmexp_mib[] = {CTL_VM, VM_UVMEXP};
|
||||||
|
size_t size;
|
||||||
|
|
||||||
|
size = sizeof(*uvmexp);
|
||||||
|
|
||||||
|
return sysctl(uvmexp_mib, 2, uvmexp, &size, NULL, 0) >= 0 ? 1 : 0;
|
||||||
|
}
|
||||||
|
|
||||||
|
const char *
|
||||||
|
ram_free(void)
|
||||||
|
{
|
||||||
|
struct uvmexp uvmexp;
|
||||||
|
float free;
|
||||||
|
int free_pages;
|
||||||
|
|
||||||
|
if (load_uvmexp(&uvmexp)) {
|
||||||
|
free_pages = uvmexp.npages - uvmexp.active;
|
||||||
|
free = (double) (free_pages * uvmexp.pagesize) / 1024 / 1024 / 1024;
|
||||||
|
return bprintf("%f", free);
|
||||||
|
}
|
||||||
|
|
||||||
|
return NULL;
|
||||||
|
}
|
||||||
|
|
||||||
|
const char *
|
||||||
|
ram_perc(void)
|
||||||
|
{
|
||||||
|
struct uvmexp uvmexp;
|
||||||
|
int percent;
|
||||||
|
|
||||||
|
if (load_uvmexp(&uvmexp)) {
|
||||||
|
percent = uvmexp.active * 100 / uvmexp.npages;
|
||||||
|
return bprintf("%d", percent);
|
||||||
|
}
|
||||||
|
|
||||||
|
return NULL;
|
||||||
|
}
|
||||||
|
|
||||||
|
const char *
|
||||||
|
ram_total(void)
|
||||||
|
{
|
||||||
|
struct uvmexp uvmexp;
|
||||||
|
float total;
|
||||||
|
|
||||||
|
if (load_uvmexp(&uvmexp)) {
|
||||||
|
total = (double) (uvmexp.npages * uvmexp.pagesize) / 1024 / 1024 / 1024;
|
||||||
|
return bprintf("%f", total);
|
||||||
|
}
|
||||||
|
|
||||||
|
return NULL;
|
||||||
|
}
|
||||||
|
|
||||||
|
const char *
|
||||||
|
ram_used(void)
|
||||||
|
{
|
||||||
|
struct uvmexp uvmexp;
|
||||||
|
float used;
|
||||||
|
|
||||||
|
if (load_uvmexp(&uvmexp)) {
|
||||||
|
used = (double) (uvmexp.active * uvmexp.pagesize) / 1024 / 1024 / 1024;
|
||||||
|
return bprintf("%f", used);
|
||||||
|
}
|
||||||
|
|
||||||
|
return NULL;
|
||||||
|
}
|
||||||
#endif
|
#endif
|
||||||
|
Reference in New Issue
Block a user